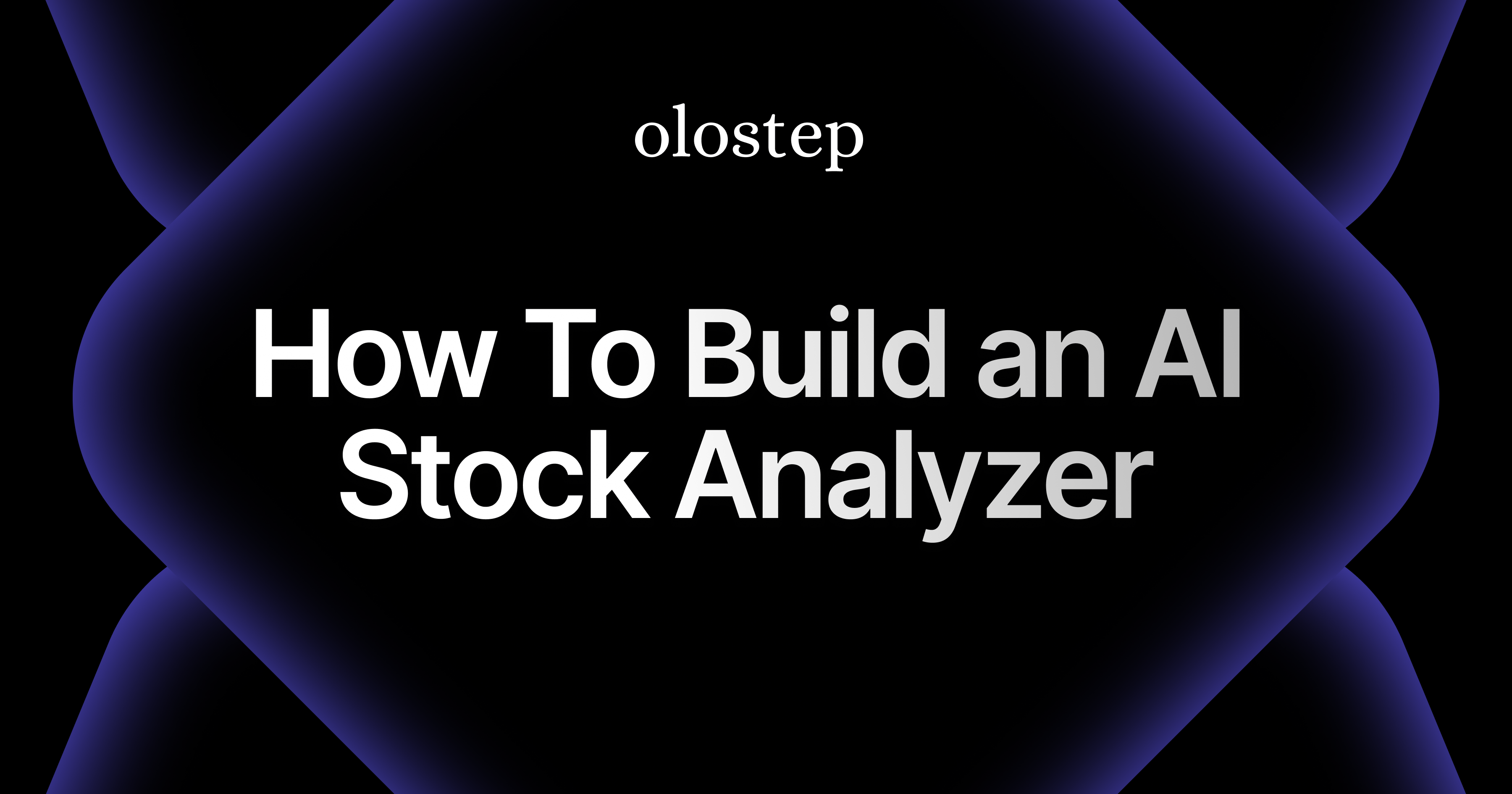
Build an AI-Powered Stock Analyzer Using Streamlit, Olostep, and OpenAI

Ehsan
Build an AI-Powered Stock Analyzer Using Streamlit, Olostep, and OpenAI.
Ever wanted to analyze stocks using AI without manually going through dozens of websites?
In this tutorial, youโll build a Streamlit app that:
- Uses Olostep's scraping API to fetch stock data from MarketWatch
- Sends the content to OpenAI GPT-4 to rate stocks based on their performance and description
- Visualizes the investment score with interactive charts
๐ ๏ธ Requirements
Make sure to install the following dependencies:
pip install streamlit openai requests python-dotenv matplotlib beautifulsoup4
Create a .env
file and add:
OPENAI_API_KEY=your_openai_key
OLOSTEP_API_KEY=your_olostep_key
๐ง Step 1: Scrape Stock Pages with Olostep
Use Olostepโs scrape
endpoint to fetch the latest data for stock tickers from MarketWatch.
def scrape_stock_page(ticker):
headers = {
"Authorization": f"Bearer {os.getenv('OLOSTEP_API_KEY')}",
"Content-Type": "application/json"
}
payload = {
"formats": ["markdown"],
"country": "US",
"url_to_scrape": f"https://www.marketwatch.com/investing/stock/{ticker}"
}
res = requests.post("https://api.olostep.com/v1/scrapes", headers=headers, json=payload)
return res.json().get("result", {}).get("markdown_content", "")
๐ง Step 2: Analyze Stock Content with OpenAI GPT-4
We ask GPT-4 to evaluate and assign a score from 0 to 100 for each stock.
def analyze_stocks_with_gpt(tickers, markdowns):
prompt = "You are a stock analyst. Based on the following markdowns from MarketWatch, rate each stock on a scale of 0โ100 as an investment opportunity. Return only JSON format like:
"
prompt += '''{
"scores": [
{"stock_name": "AAPL", "score": 92},
{"stock_name": "MSFT", "score": 88}
]
}'''
for ticker, md in zip(tickers, markdowns):
prompt += f"Ticker: {ticker}
Content:
{md[:3000]}
"
response = openai_client.chat.completions.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}],
)
return json.loads(response.choices[0].message.content.strip())
๐จ Step 3: Visualize Results in Streamlit
def plot_scores(scores):
stock_names = [item['stock_name'] for item in scores]
values = [item['score'] for item in scores]
fig, ax = plt.subplots(figsize=(10, 4))
ax.bar(stock_names, values, color="green")
ax.set_ylabel("Investment Score")
ax.set_title("AI-Scored Stocks by OpenAI GPT-4")
st.pyplot(fig)
๐ Step 4: Complete Streamlit App
st.set_page_config(page_title="AI Stock Analyzer", page_icon="๐")
st.title("๐ AI Stock Analyzer with Olostep + OpenAI")
tickers_input = st.text_input("Enter comma-separated stock tickers (e.g., AAPL,MSFT,TSLA)")
if st.button("Analyze"):
tickers = [t.strip().lower() for t in tickers_input.split(",") if t.strip()]
markdowns = [scrape_stock_page(ticker) for ticker in tickers]
if not any(markdowns):
st.error("No data found.")
else:
st.info("Analyzing stocks with GPT...")
scores = analyze_stocks_with_gpt(tickers, markdowns)
st.success("Analysis complete!")
plot_scores(scores['scores'])
โ Example Output
Stock | Score |
---|---|
AAPL | 91 |
TSLA | 84 |
MSFT | 89 |
๐ผ Use Cases
- Retail investors screening multiple stocks
- Analysts comparing high-volume tickers
- Developers building AI-based investing tools
- Newsletter writers looking to automate stock ideas
๐ฎ What's Next
- Export scores to a Google Sheet or PDF
- Use historical stock data and financial ratios
- Add GPT explanation for each score
- Deploy on Streamlit Cloud
๐ก Final Thoughts
This app combines real-time scraping, AI rating, and visual insights into one seamless experience. Itโs ideal for anyone looking to use AI to make smarter investment decisions at scale.
Happy investing! ๐